Calendar View
What is an API End points
An API endpoint is a point at which an API (the code that allows two software programs to communicate with each other) connects with the software program. APIs work by sending requests for information from a web application or web server and receiving a response.
How API endpoints work
One side sends the information to the API and is called the server. The other side, the client, makes the requests and manipulates the API. The server side that provides the requested information, or resources, is the API endpoint.
Project container structure
In our case the client is going to be Student, Mentor and Admin as seen in the class diagram.
API connection
After creating the API locally, it will be hosted on an api hosting platform. Swagger is not only good for documenting the API but also has features for running and testing the API. The API should be able to respond to requests as seen below.
When making GET request to the link, the API responds with the following JSON data
[Base URL: scholarsacademyprojectapi.com/v1]
Admin
request = GET /admin/login
GET /admin/login Logs admin into the system
Parameters
Name |
Description |
---|---|
username(required) |
|
string |
The user name used for login |
password(required) |
|
string |
The password used for login |
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
400 |
Invalid username/password |
{
"code": 200,
"request": "admin_login",
"message": "Successfully Logged In!"
}
{
"code": 400,
"request": "admin_login",
"message": "Invalid username or password. Please check try again"
}
request = GET /admin/getCalendarView
GET /admin/getCalendarView Gets the Calendar data
Parameters
No Parameters needed.
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
{
"name":"Dinaol Tadesse",
"courses":[
"csc 118",
"calculus"
],
"calendar":[
{
"weeks":[
"Tuesday",
"Thursday"
],
"startTime":"00:12:00",
"endTime":"00:13:00"
},
{
"weeks":[
"Monday",
"Wednesday"
],
"startTime":"00:07:00",
"endTime":"00:09:00"
}
]
}
request: POST /admin/addMentor
Adds New Mentor to Database
Parameters
Name |
Description |
---|---|
body(required) |
|
object |
New Mentor Object. |
Example body value:
{
"name":"Dinaol Tadesse",
"courses":[
"csc 118",
"calculus"
],
"calendar":[
{
"weeks":[
"Tuesday",
"Thursday"
],
"startTime":"00:12:00",
"endTime":"00:13:00"
},
{
"weeks":[
"Monday",
"Wednesday"
],
"startTime":"00:07:00",
"endTime":"00:09:00"
}
]
}
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
400 |
Invalid Schedule supplied |
request: PUT /admin/changeSchedule
Updates the Schedule of Existing Mentor
Parameters
Name |
Description |
---|---|
jNumber(required) |
|
Int |
The J number of the Mentor User |
body(required) |
|
object |
Updated user Object. |
Example body value:
{
"name":"Dinaol Tadesse",
"courses":[
"CSC 118",
"Data Structures"
],
"calendar":[
{
"weeks":[
"Tuesday",
"Thursday"
],
"startTime":"00:12:00",
"endTime":"00:13:00"
},
{
"weeks":[
"Monday",
"Wednesday"
],
"startTime":"00:07:00",
"endTime":"00:09:00"
}
]
}
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
400 |
Invalid Schedule supplied |
request: GET /admin/logout
request: GET /admin/logout Logs out the admin
Parameters
No Parameters needed.
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
{
"code": 200,
"request": "admin_logout",
"message": "Logged Out"
}
Mentor
request: GET /mentor/login
Logs Mentor into the system
Parameters
Name |
Description |
---|---|
username(required) |
|
string |
The user name used for login |
password(required) |
|
string |
The password used for login |
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
400 |
Invalid username/password |
{
"code": 200,
"request": "mentor_login",
"message": "Successfully Logged In!"
}
{
"code": 400,
"request": "mentor_login",
"message": "Invalid username or password. Please check try again"
}
request: GET /mentor/getCalendarView
the Calendar data
Parameters
No Parameters needed.
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
{
"name":"Dinaol Tadesse",
"courses":[
"csc 118",
"calculus"
],
"calendar":[
{
"weeks":[
"Tuesday",
"Thursday"
],
"startTime":"00:12:00",
"endTime":"00:13:00"
},
{
"weeks":[
"Monday",
"Wednesday"
],
"startTime":"00:07:00",
"endTime":"00:09:00"
}
]
}
request: PUT /mentor/changeSchedule
Updates Schedule of the Mentor
Parameters
Name |
Description |
---|---|
body(required) |
|
object |
Updated user Object. |
Example body value:
{
"name":"Dinaol Tadesse",
"courses":[
"csc 118",
"calculus"
],
"calendar":[
{
"weeks":[
"Tuesday",
"Thursday"
],
"startTime":"00:12:00",
"endTime":"00:13:00"
},
{
"weeks":[
"Monday",
"Wednesday"
],
"startTime":"00:07:00",
"endTime":"00:09:00"
}
]
}
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
400 |
Invalid Schedule supplied |
request: GET /mentor/logout
Logs out the Mentor
Parameters
No Parameters needed.
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
{
"code": 200,
"request": "mentor_logout",
"message": "Logged Out"
}
Student
request: GET /student/login
Logs Student into the system
Parameters
Name |
Description |
---|---|
username(required) |
|
string |
The user name used for login |
password(required) |
|
string |
The password used for login |
Responses
Code |
Description |
---|---|
200 |
Successful Operation! |
400 |
Invalid username/password |
{
"code": 200,
"request": "student_login",
"message": "Successfully Logged In!"
}
{
"code": 400,
"request": "student_login",
"message": "Invalid username or password. Please check try again"
}
request: GET /student/getCalendarView
Gets the Calendar Data.
Parameters
No Parameters needed.
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
{
"name":"Dinaol Tadesse",
"courses":[
"csc 118",
"calculus"
],
"calendar":[
{
"weeks":[
"Tuesday",
"Thursday"
],
"startTime":"00:12:00",
"endTime":"00:13:00"
},
{
"weeks":[
"Monday",
"Wednesday"
],
"startTime":"00:07:00",
"endTime":"00:09:00"
}
]
}
request: POST /student/scheduleEvent
create appointment with Mentor
Parameters
Name |
Description |
---|---|
body(required) |
|
object |
New event object. |
Example body value:
{
"eventId": 12,
"mentorName": "Dinaol Tadesse",
"studentName": "Amber Heard",
"startDate": "2022-05-23 T21:15:23",
"endDate": "2022-05-23 T22:15:23"
}
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
400 |
Invalid Event supplied |
404 |
Event not found |
request: GET /student/logout
Logs out the Student
Parameters
No Parameters needed.
Responses
Code |
Description |
---|---|
200 |
Successful Operation |
{
"code": 200,
"request": "Student_logout",
"message": "Logged Out"
}
Calendar UI
For displaying the calendar on our application, there are different react calendar libraries. We can use the React Big Calendar library. A small demo of how the calendar looks like can be found here.
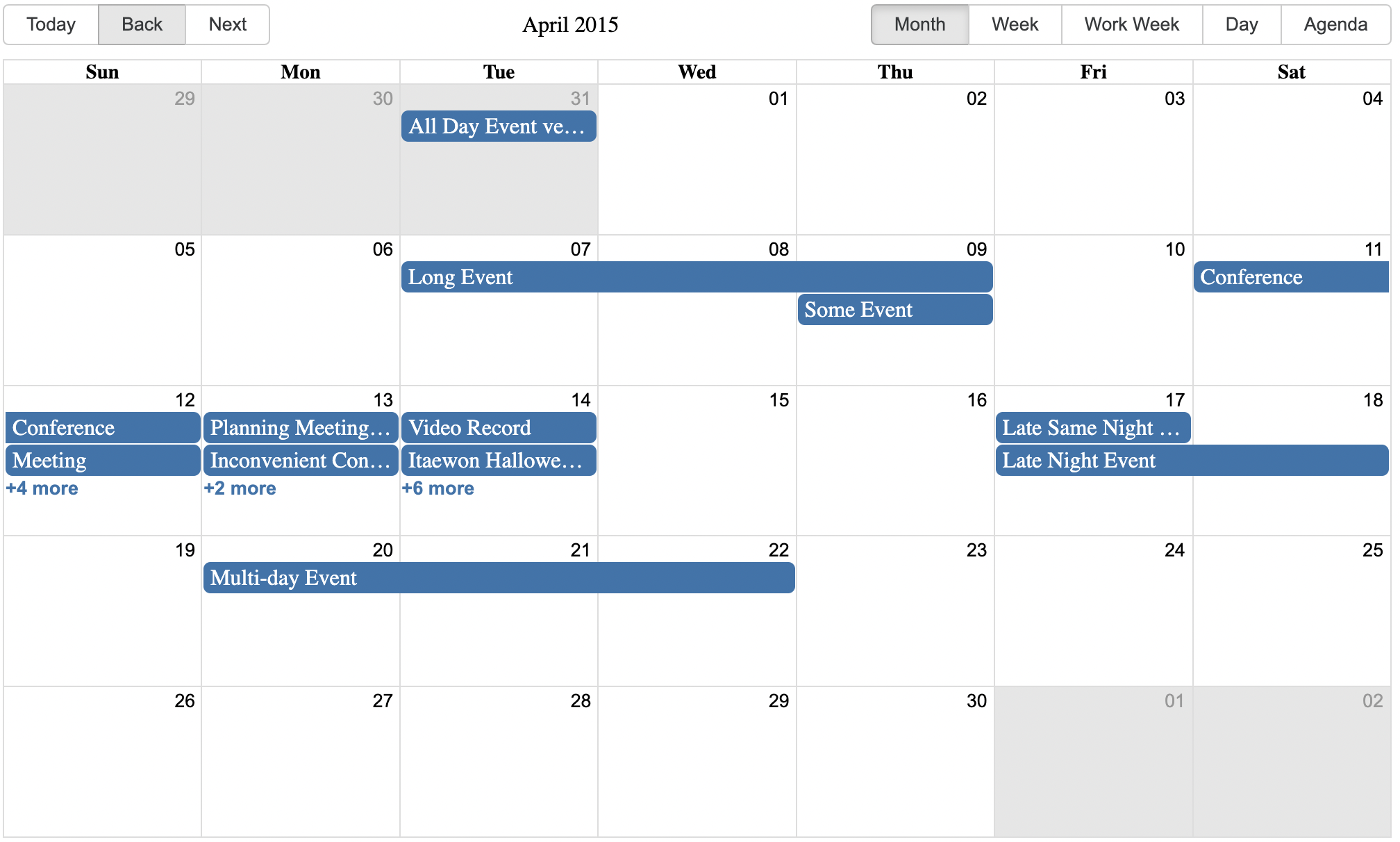
Screen Shot 2022-05-18 at 9.21.24 PM.png
Resources
OpenAPI - standard way to describe an API
Swagger API - https://swagger.io/specification/
Amazon API gateway
Requestbin: https://pipedream.com/workflows
API Creation: https://blog.stoplight.io/how-to-create-an-api-in-three-steps